How to Use sh for Basic Shell Scripting: A Beginner's Guide
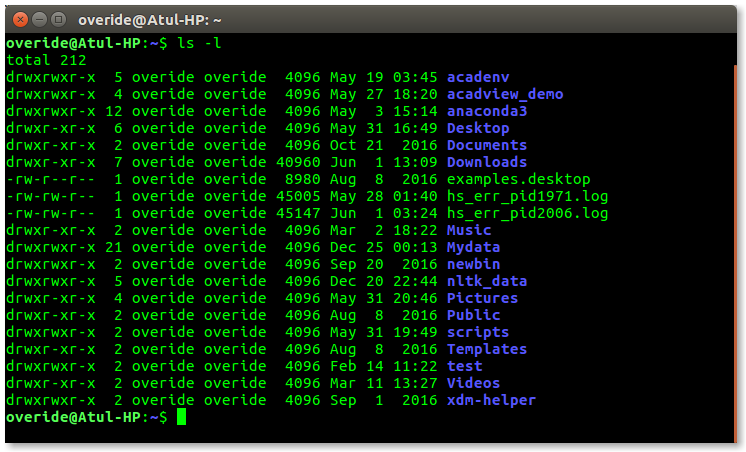
How to Use sh
for Basic Shell Scripting: A Beginner’s Guide
Shell scripting is a powerful tool in Unix-like operating systems that allows users to automate repetitive tasks, perform complex operations, and manage systems more efficiently. The sh
shell, often referred to as the Bourne shell, is one of the oldest and simplest shell environments used for basic scripting. This guide will walk you through the basics of shell scripting using sh
, providing examples and best practices along the way.
Whether you’re a system administrator, developer, or Linux enthusiast, learning shell scripting will enhance your ability to automate tasks and simplify command-line workflows.
What is Shell Scripting?
A shell script is essentially a text file containing a series of commands that the shell interprets and executes. It’s a program written for the shell, a command-line interpreter that allows you to interact with your operating system.
The sh
shell, specifically, is widely available on most Unix-based systems and serves as the foundation for many other shells like bash
and dash
. Using sh
for shell scripting offers a basic and portable way to write scripts that can run across different systems.
Step 1: Writing Your First Shell Script
Creating a Shell Script File
To begin writing a shell script, you’ll need a text editor and a terminal. Popular text editors like Vim, Nano, or even graphical ones like Visual Studio Code can be used to write your script.
Let’s create a simple shell script:
- Open your terminal.
- Create a new file with a
.sh
extension, which indicates a shell script. For example:
touch myscript.sh
Open this file in a text editor. If you’re using Nano, you can do this with: