How to Use Docker to Deploy Node.js Applications
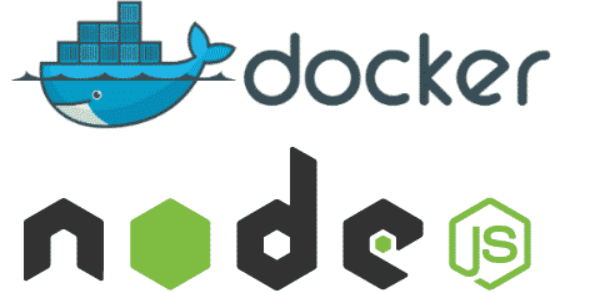
How to Use Docker to Deploy Node.js Applications
In the realm of modern web development, Docker has emerged as an invaluable tool for deploying applications. It allows developers to package applications and their dependencies into containers, ensuring consistency across different environments. This article will guide you through the steps to deploy Node.js applications using Docker, providing a comprehensive understanding of the process.
What is Docker?
Docker is a platform designed to automate the deployment of applications within lightweight, portable containers. These containers encapsulate an application and its environment, making it easy to run the application on any system that supports Docker. This abstraction simplifies many of the issues developers face when deploying applications, such as dependencies and environment configurations.
Why Use Docker for Node.js Applications?
Consistency Across Environments: Docker ensures that your application runs the same way in development, testing, and production environments. This minimizes the “it works on my machine” problem.
- Isolation: Each Docker container runs in its own environment, preventing conflicts between different applications or versions of Node.js.
- Scalability: Docker makes it easy to scale applications by allowing you to run multiple instances of a container.
- Portability: Docker containers can run on any system that has Docker installed, making it easy to move applications between environments.
Prerequisites
Before diving into the deployment process, ensure you have the following installed:
- Docker: You can download Docker from Docker’s official website.
- Node.js: Install Node.js from the Node.js website.
Step 1: Create a Simple Node.js Application
Let’s start by creating a simple Node.js application. Create a directory for your project and navigate to it:
mkdir my-node-app
cd my-node-app
Next, initialize a new Node.js application:
npm init -y
This command will generate a package.json file. Now, create a file named app.js and add the following code:
const express = require(‘express’);
const app = express();
const port = process.env.PORT || 3000;app.get(‘/’, (req, res) => {
res.send(‘Hello World! This is my Node.js application running in Docker.’);
});app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
You will also need to install Express, so run:
npm install express
Step 2: Create a Dockerfile
A Dockerfile is a text document that contains all the commands to assemble an image. In your project directory, create a file named Dockerfile without any extension and add the following content:
# Use the official Node.js image as a base
FROM node:14
# Set the working directory in the container
WORKDIR /usr/src/app
# Copy package.json and package-lock.json to the working directory
COPY package*.json ./
# Install the dependencies
RUN npm install
# Copy the rest of the application code
COPY . .
# Expose the port that the app runs on
EXPOSE 3000
# Command to run the application
CMD [“node”, “app.js”]
Explanation of the Dockerfile:
FROM node:14: This line specifies the base image for the container, which in this case is the official Node.js image with version 14.
WORKDIR /usr/src/app: Sets the working directory inside the container.
*COPY package.json ./**: Copies the package.json and package-lock.json files to the container.
RUN npm install: Installs the application dependencies.
COPY . .: Copies the rest of the application code into the container.
EXPOSE 3000: Informs Docker that the container listens on port 3000 at runtime.
CMD [“node”, “app.js”]: Specifies the command to run the application.
Step 3: Build the Docker Image
Now that you have a Dockerfile, it’s time to build your Docker image. In your terminal, run the following command from the directory containing your Dockerfile:
docker build -t my-node-app .
This command tells Docker to build an image named my-node-app using the current directory (denoted by the .) as the context.
Step 4: Run the Docker Container
After the image has been built successfully, you can run it with the following command:
docker run -p 3000:3000 my-node-app
This command maps port 3000 of your host machine to port 3000 of the container, allowing you to access your application from the host.
Step 5: Access the Application
Once the container is running, open your web browser and go to http://localhost:3000. You should see the message “Hello World! This is my Node.js application running in Docker.”
Step 6: Managing Your Docker Containers
To view running containers, use the command:
docker ps
To stop the container, find the container ID from the docker ps command and run:
docker stop
node.js Official Documentation
Thank you for visiting our page! Don’t forget to check out our other article through the link below to enhance your Linux skills. Also, be sure to read our guide on How to Set Up a Minecraft Server with Docker on Ubuntu! 🙂