How to Set Up Git Hooks for Automated Tasks
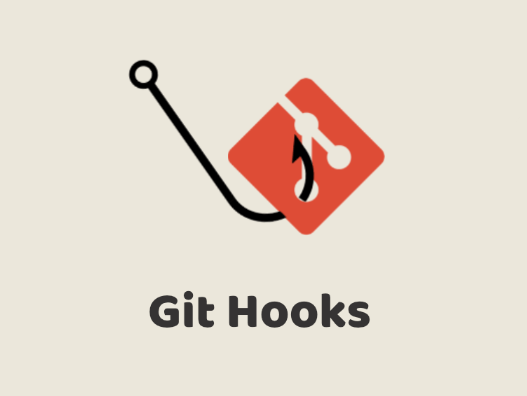
How to Set Up Git Hooks for Automated Task
Introduction
Git is a powerful version control system that developers worldwide use to manage and track changes in their projects. One of the lesser-known but incredibly useful features of Git is Git hooks. Git hooks allow you to automate tasks by running scripts at different stages of your Git workflow. This can save time, enforce coding standards, and streamline your development process. In this guide, we will explore how to set up Git hooks for automated tasks, the types of hooks available, and provide examples to get you started.
What Are Git Hooks?
Git hooks are scripts that run automatically when certain events occur in a Git repository. For instance, you can set up a hook to run tests before committing changes or to deploy code after pushing it to a remote repository. Git hooks are stored in the .git/hooks directory of your repository, and they can be customized to suit your workflow needs.
There are two main types of Git hooks:
- Client-Side Hooks – These run on operations like committing, merging, and checking out code.
- Server-Side Hooks – These execute on actions like pushing or receiving commits on the server.
Why Use Git Hooks?
Before diving into how to set up Git hooks, it’s important to understand why they are beneficial:
- Automate Tasks: Git hooks can automate repetitive tasks, such as running tests or formatting code.
- Enforce Code Quality: Ensure that code passes certain checks before being committed or pushed.
- Streamline Workflow: Speed up the development process by automating mundane tasks.
- Prevent Mistakes: Reduce the chances of pushing incomplete or buggy code by setting up pre-commit or pre-push hooks.
How to Set Up Git Hooks
Setting up Git hooks is straightforward. Let’s go step by step:
Step 1: Access the Git Hooks Directory
Navigate to the .git/hooks directory in your local repository. You can do this by running:
cd /path/to/your/repository/.git/hooks
Inside this directory, you will find sample hook scripts (e.g., pre-commit.sample, post-commit.sample). These are disabled by default, but they can serve as a great starting point for creating your own hooks.
Step 2: Create or Modify a Hook Script
To set up a new hook, create a new file with the name of the desired hook (e.g., pre-commit, post-merge). Make sure the file is executable. For example:
touch pre-commit
chmod +x pre-commit
Open the pre-commit file and add the following script to run tests before committing:
#!/bin/bash
# Run tests before committing
echo “Running tests…”
npm test# If tests fail, abort the commit
if [ $? -ne 0 ]; then
echo “Tests failed. Commit aborted.”
exit 1
fiecho “Tests passed. Proceeding with commit.”
Step 3: Test the Hook
Make a change to your code and try to commit it. If the hook is set up correctly, the script will run before the commit. If the tests fail, the commit will be aborted, and you will see an error message.
Types of Git Hooks
Here’s a quick overview of some common Git hooks and how you can use them:
- Pre-Commit – Runs before you make a commit. This is often used to check code for errors, run tests, or format code.
- Commit-Msg – Used to validate the commit message. You can enforce rules on the commit message, such as requiring a certain format.
- Pre-Push – Runs before you push to a remote repository. This can be used to run tests, check for merge conflicts, or validate other aspects of the codebase.
- Post-Commit – Executes after a commit has been made. This can be used for tasks like updating a log or triggering notifications.
- Pre-Rebase – Runs before you start a rebase operation. You might use this to ensure that no commits are in progress or to back up your work.
Example Use Cases
Let’s go through a few examples of how you might use Git hooks in your workflow:
1. Running Linting on Pre-Commit
Make sure your code meets style guidelines before committing:
#!/bin/bash
# Run ESLint before committing
echo “Running ESLint…”
eslint .if [ $? -ne 0 ]; then
echo “ESLint errors detected. Commit aborted.”
exit 1
fiecho “ESLint passed. Proceeding with commit.”
2. Enforcing Commit Message Format
Ensure that commit messages follow a specific format (e.g., include a ticket number):
#!/bin/bash
# Enforce commit message format
message=$(cat $1)if ! [[ $message =~ ^[A-Z]{2,}-[0-9]+: ]]; then
echo “Error: Commit message must start with a ticket number (e.g., ABC-123: Description).”
exit 1
fi
3. Pre-Push Hook to Run Tests
Make sure all tests pass before allowing a push to the remote repository:
#!/bin/bash
echo “Running tests before push…”
npm testif [ $? -ne 0 ]; then
echo “Tests failed. Push aborted.”
exit 1
fiecho “All tests passed. Proceeding with push.”
Best Practices for Using Git Hooks
- Keep Hooks Simple – If your hooks are complex, consider creating a separate script file and call it from the hook. This keeps the .git/hooks directory clean and manageable.
- Use Hook Libraries – Libraries like Husky can simplify managing Git hooks, especially in larger projects.
- Share Hooks Across Team – Hooks are not version-controlled by default. To share them with your team, you can create a separate directory (e.g., .githooks) and add it to version control. Use a Git configuration to specify the path:
git config core.hooksPath .githooks
Thank you for visiting our page! 🙂 You can click on the link below to familiarize yourself with Linux systems and ‘How to Use VirtualBox to Create Virtual Machines on Linux’ to read our article. Good luck!