How to Set Up a Git Workflow for Development
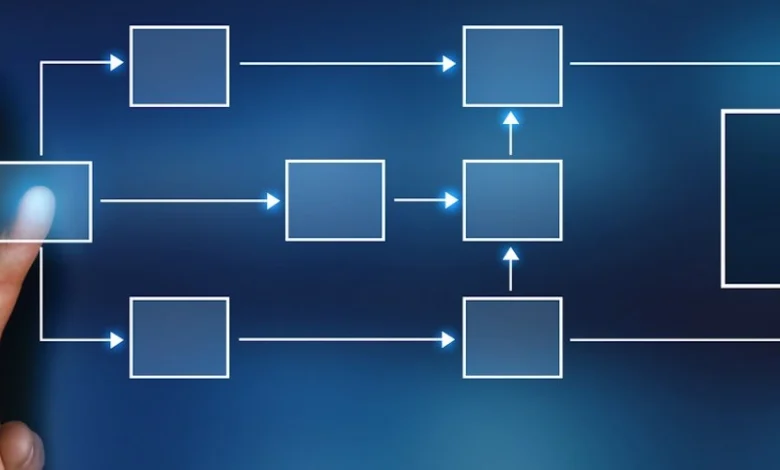
A proper Git workflow is essential for effective collaboration and code management in development projects. This guide covers setting up a standard Git workflow, including detailed explanations, commands, and best practices.
What is Git?
Git is a distributed version control system used for tracking changes in source code during software development. It enables multiple developers to work on a project simultaneously while maintaining a complete history of modifications.
Why Use a Git Workflow?
A Git workflow provides a structured approach to collaboration. Benefits include:
- Efficient Collaboration: Multiple developers can work on different features without interference.
- Version Control: Ability to track changes and revert when necessary.
- Consistency: Standardized processes ensure smooth collaboration and integration.
Step 1: Initialize a Git Repository
To start a new Git project, create a directory and initialize it:
mkdir my-project
cd my-project
git init
This initializes a new Git repository in the my-project
directory, preparing it for version control.
Step 2: Clone an Existing Repository
If you need to work on an existing project hosted on a remote server:
git clone [repository-url]
This clones the repository into a new directory on your local machine.
Step 3: Configuring User Information
Ensure your Git user details are set correctly:
git config --global user.name "Your Name"
git config --global user.email "[email protected]"
Step 4: Branching Strategy
Branching allows you to work on new features without affecting the main codebase. A common strategy involves the following branches:
main
: The stable production branch.develop
: The primary development branch.feature/*
: Feature-specific branches.hotfix/*
: For emergency fixes.
To create and switch to a new branch:
git checkout -b feature-branch
Step 5: Making Changes and Committing
Modify your code and prepare to commit changes:
git add .
git commit -m "Description of the changes made"
Step 6: Pushing Changes to Remote Repository
To share your changes with others:
git push origin feature-branch
Step 7: Pull Requests and Code Reviews
Open a pull request (PR) on platforms like GitHub or GitLab. Reviewers can provide feedback before merging changes.
Step 8: Merging Changes
After approval, merge the changes into the main
branch:
git checkout main
git merge feature-branch
git push origin main
Step 9: Handling Merge Conflicts
Conflicts occur when changes from different branches overlap. Resolve them by manually editing conflicting files, then:
git add .
git commit -m "Resolved merge conflicts"
Step 10: Syncing with the Remote Repository
Ensure your local repository is up to date:
git pull origin main
Advanced Git Features
Using Tags
Tags mark important milestones or versions in a project:
git tag v1.0.0
git push origin v1.0.0
Rebasing
Rebasing rewrites commit history for cleaner collaboration:
git rebase main
Best Practices
- Commit Frequently: Break changes into small, logical commits.
- Write Clear Messages: Describe changes clearly in commit messages.
- Avoid Large Pull Requests: Keep PRs focused on a single feature or fix.
- Backup Work: Use remote repositories to back up code.
- Document the Workflow: Ensure all team members follow the same workflow.
Common Git Commands Cheat Sheet
git status
: Check current branch and changes.git log
: View commit history.git revert
: Undo a commit.git stash
: Temporarily save changes without committing.git reset
: Undo changes in the working directory.
Troubleshooting Git Issues
- Detached HEAD State: Ensure you’re on a branch using
git checkout main
. - Permission Denied: Check your SSH keys and repository permissions.
- Outdated Local Repo: Use
git pull
to sync with the remote repository.
Conclusion
A well-defined Git workflow enhances collaboration, ensures code quality, and simplifies project management. By mastering branching strategies, committing best practices, and advanced features like tagging and rebasing, development teams can streamline their version control processes for maximum efficiency.