How to Install and Use SASS for CSS Preprocessing
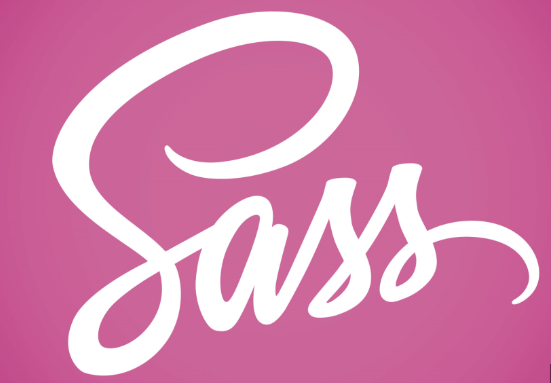
How to Install and Use SASS for CSS Preprocessing
Introduction
In modern web development, styling plays a crucial role in creating visually appealing and user-friendly websites. CSS, or Cascading Style Sheets, is the standard language used for styling web pages. However, managing large CSS files can be cumbersome and challenging. This is where SASS (Syntactically Awesome Style Sheets) comes into play. SASS is a CSS preprocessor that adds power and elegance to the basic language, allowing you to write clean, maintainable, and reusable code.
In this guide, we’ll walk you through what SASS is, how to install it, and how to use it to make your CSS workflow more efficient.
What is SASS?
SASS is a CSS preprocessor that extends the functionality of CSS by introducing features like variables, nested rules, mixins, and more. It helps in writing more maintainable, readable, and reusable code, making it easier to handle large projects. By compiling SASS into standard CSS, you can use all its advanced features without worrying about browser compatibility.
Benefits of Using SASS
- Variables: Store values you want to reuse throughout your stylesheets.
- Nesting: Nest your CSS selectors in a way that follows the same visual hierarchy of your HTML.
- Partials and Import: Break down your CSS into smaller, manageable chunks and import them.
- Mixins: Create reusable pieces of code that can be reused throughout your CSS files.
- Inheritance: Share a set of CSS properties from one selector to another.
These features not only make writing CSS quicker but also help maintain clean and organized code.
Prerequisites
Before you begin, ensure you have the following:
- Node.js and npm: SASS can be installed via npm (Node Package Manager), so you need Node.js installed on your system.
- Command Line Basics: Familiarity with using the command line or terminal.
Step 1: Installing Node.js and npm
If you don’t already have Node.js installed, follow these steps:
Go to the Node.js website and download the installer.
Run the installer and follow the instructions to complete the installation.
After installation, open your terminal (Command Prompt, PowerShell, or Terminal) and type:
node -v
npm -v
This will display the installed versions of Node.js and npm, confirming they are successfully installed.
Step 2: Installing SASS
Once Node.js is installed, you can install SASS globally on your system using npm. Open your terminal and run:
npm install -g sass
The -g flag ensures that SASS is installed globally, making it accessible from any directory on your system. After the installation, verify by running:
sass –version
You should see the installed version number, which confirms that SASS is installed successfully.
Step 3: Using SASS in Your Project
1. Creating Your SASS File
Create a new .scss file (SASS uses the .scss file extension) in your project directory. For example:
styles.scss
2. Writing SASS Code
Let’s say you want to define some variables and mixins. Here’s a basic example:
// Define variables
$primary-color: #3498db;
$font-stack: Helvetica, sans-serif;// Create a mixin
@mixin border-radius($radius) {
-webkit-border-radius: $radius;
-moz-border-radius: $radius;
border-radius: $radius;
}// Use variables and mixins
body {
font: 100% $font-stack;
color: $primary-color;
}button {
@include border-radius(5px);
}
3. Compiling SASS to CSS
You need to compile the SASS file into a CSS file for it to be used on your web pages. To do this, run:
sass styles.scss styles.css
This command will compile styles.scss into styles.css. You can also watch for changes and automatically recompile the file by using:
sass –watch styles.scss:styles.css
This command will monitor styles.scss for any changes and update styles.css automatically.
Step 4: Advanced SASS Features
1. Nesting
SASS allows you to nest your CSS rules, making your code more organized:
nav {
ul {
list-style: none;
}li {
display: inline-block;
}a {
text-decoration: none;
color: $primary-color;
}
}
2. Partials and Import
Partials are smaller SASS files that can be imported into other files. This is helpful for breaking down your CSS:
// _variables.scss
$primary-color: #3498db;
$font-stack: Helvetica, sans-serif;// _mixins.scss
@mixin box-shadow($x, $y, $blur, $color) {
-webkit-box-shadow: $x $y $blur $color;
-moz-box-shadow: $x $y $blur $color;
box-shadow: $x $y $blur $color;
}
You can import these partials into your main .scss file:
@import ‘variables’;
@import ‘mixins’;
3. Inheritance/Extend
Instead of duplicating code, you can extend existing styles:
.btn {
padding: 10px 20px;
background-color: $primary-color;
color: white;
}.btn-primary {
@extend .btn;
background-color: darken($primary-color, 10%);
}
Step 5: Using SASS in Production
When you are ready to move your SASS code into production, you should compress your CSS to reduce file size. To do this, run:
sass styles.scss styles.min.css –style compressed
This will output a minified version of your CSS, making it faster for users to download.
Conclusion
SASS is an incredibly powerful tool that streamlines the process of writing and maintaining CSS. By using variables, mixins, nesting, and more, you can keep your stylesheets clean, organized, and scalable. Whether you’re working on a small website or a large web application, SASS can save you time and effort, allowing you to focus more on creating beautiful designs.
Start using SASS today, and experience a more efficient way to handle your CSS.