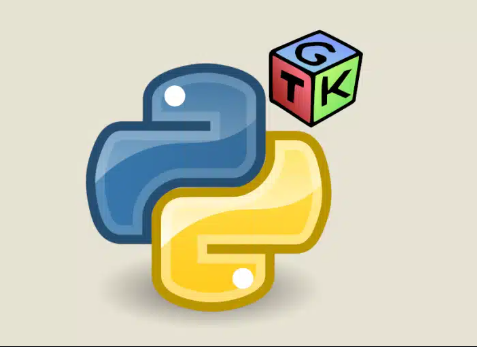
How to Install and Use PyGTK for GUI Development
Creating graphical user interfaces (GUIs) in Python can be efficiently done using PyGTK, a set of Python wrappers for the GTK (GIMP Toolkit) libraries. This guide will walk you through the installation process and provide you with a comprehensive understanding of how to utilize PyGTK for your GUI development projects.
What is PyGTK?
PyGTK allows developers to create applications with a native look and feel on various operating systems such as Linux, Windows, and macOS. It provides a wide range of widgets (buttons, labels, text boxes, etc.) that help in building sophisticated interfaces. The beauty of using PyGTK lies in its ability to integrate seamlessly with Python, making it easier for developers to write readable and maintainable code.
Installing PyGTK
Before you start building applications with PyGTK, you need to install it. The installation process varies slightly depending on your operating system.
On Ubuntu or Debian-based Systems
Open a terminal window. You can find the terminal in your applications menu.
Update your package list:
sudo apt update
Install the PyGTK package:
sudo apt install python3-gi python3-gi-cairo gir1.2-gtk-3.0
- Verify the installation: You can check if PyGTK is installed correctly by running a simple Python script:
import gi
gi.require_version(‘Gtk’, ‘3.0’)
from gi.repository import Gtkprint(“PyGTK is installed correctly!”)
On Windows
Installing PyGTK on Windows is a bit different since you need to download the binaries and set them up manually.
Download the GTK installer from the GTK official website.
Run the installer and follow the prompts to complete the installation.
Set up the environment variable:
- Search for “Environment Variables” in the Windows search bar.
- Click on “Edit the system environment variables”.
- Under System variables, find and select the “Path” variable, then click on “Edit”.
- Click “New” and add the path where GTK is installed (usually C:\Program Files\GTK3-Runtime Win64\bin).
- Install PyGObject using pip: Open Command Prompt and run:
pip install PyGObject
Test the installation as previously described for Ubuntu.
On macOS
For macOS, you can use Homebrew to install GTK and PyGTK.
Open the Terminal.
Install Homebrew (if you haven’t already) by running:
/bin/bash -c “$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)”
Install GTK:
brew install gtk+3
Install PyGObject:
pip install PyGObject
Verify the installation by running the same Python script mentioned above.
Building Your First GUI Application
Now that you have PyGTK installed, let’s create a simple GUI application to familiarize yourself with its functionalities.
Basic Application Structure
Here’s a simple example of a PyGTK application that creates a window with a button:
import gi
gi.require_version(‘Gtk’, ‘3.0’)
from gi.repository import Gtkclass MyWindow(Gtk.Window):
def __init__(self):
super().__init__(title=”My First PyGTK App”)
self.set_size_request(400, 300)self.button = Gtk.Button(label=”Click Me”)
self.button.connect(“clicked”, self.on_button_clicked)
self.add(self.button)def on_button_clicked(self, widget):
print(“Button Clicked!”)win = MyWindow()
win.connect(“destroy”, Gtk.main_quit)
win.show_all()
Gtk.main()
Explanation of the Code
Import Libraries: You need to import the necessary modules from the gi.repository.
- Create a Window: The MyWindow class inherits from Gtk.Window, creating a new window.
- Add a Button: A button labeled “Click Me” is created and connected to a callback function, on_button_clicked, which is triggered when the button is clicked.
- Run the Application: Finally, the application enters the GTK main loop, displaying the window.
Running Your Application
Save your code in a file named my_first_app.py and run it from the terminal or command prompt:
python3 my_first_app.py
You should see a window pop up with a button. Clicking the button will print “Button Clicked!” to the terminal.
This tutorial is good, but stands the risk of confusing beginners.
The code in your example does not work in the way it is presented. It has to be correctly indented, as shown below.
import gi
gi.require_version(‘Gtk’, ‘3.0’)
from gi.repository import Gtk
class MyWindow(Gtk.Window):
def __init__(self):
super().__init__(title=”My First PyGTK App”)
self.set_size_request(400, 300)
self.button = Gtk.Button(label=”Click Me”)
self.button.connect(“clicked”, self.on_button_clicked)
self.add(self.button)
def on_button_clicked(self, widget):
print(“Button Clicked!”)
win = MyWindow()
win.connect(“destroy”, Gtk.main_quit)
win.show_all()
Gtk.main()
My bad. The formatting was not preserved. So, please fix the indentation.