How to Install and Use Terraform for Infrastructure as Code
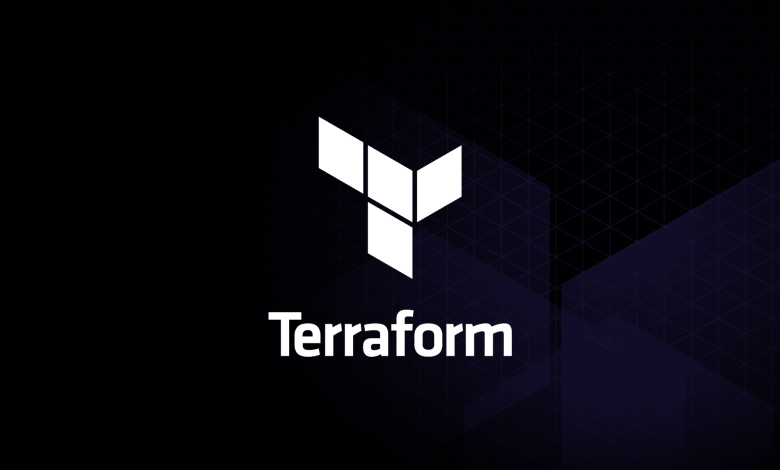
Terraform, an open-source tool by HashiCorp, allows you to define and provision infrastructure using a high-level configuration language. It is widely used for managing resources across multiple cloud providers and on-premises systems. This guide provides a step-by-step approach to installing Terraform and using it effectively for infrastructure as code (IaC).
What is Terraform?
Terraform enables you to manage infrastructure using a declarative configuration language called HashiCorp Configuration Language (HCL). It provides a unified approach to managing cloud services, networks, virtual machines, and more. Terraform’s key features include:
- Infrastructure as Code: Define your infrastructure in code files.
- Execution Plans: Preview changes before applying them.
- Resource Graph: Visualize resource dependencies.
- Automation: Reuse configurations to manage your infrastructure efficiently.
Installing Terraform
To install Terraform, follow these steps based on your operating system.
1. Download Terraform
Visit the official Terraform download page and choose the appropriate version for your operating system.
2. Install on Linux
- Download the binary:
wget https://releases.hashicorp.com/terraform/[VERSION]/terraform_[VERSION]_linux_amd64.zip
Replace
[VERSION]
with the desired version number. - Unzip the binary:
unzip terraform_[VERSION]_linux_amd64.zip
- Move the binary to
/usr/local/bin
for global access:sudo mv terraform /usr/local/bin/
- Verify the installation:
terraform -v
3. Install on macOS
- Use Homebrew:
brew install terraform
- Verify the installation:
terraform -v
4. Install on Windows
- Download the binary and unzip it to a directory of your choice.
- Add the directory to your PATH environment variable:
- Open System Properties > Advanced > Environment Variables.
- Edit the
Path
variable and add the directory containingterraform.exe
.
- Verify the installation:
terraform -v
Getting Started with Terraform
1. Initialize a Terraform Project
- Create a working directory:
mkdir terraform_project cd terraform_project
- Create a configuration file: Write your infrastructure code in a file named
main.tf
.Example for an AWS EC2 instance:
provider "aws" { region = "us-east-1" } resource "aws_instance" "example" { ami = "ami-0c55b159cbfafe1f0" instance_type = "t2.micro" tags = { Name = "TerraformExample" } }
- Initialize Terraform:
terraform init
This command downloads necessary plugins and prepares the working directory.
2. Plan Infrastructure Changes
Run the plan
command to preview changes:
terraform plan
This step ensures you understand the impact of the changes before applying them.
3. Apply Changes
Apply the changes to create resources:
terraform apply
Type yes
when prompted to confirm.
4. Verify the Infrastructure
Log in to your cloud provider’s console to verify the created resources. For the above example, check the AWS EC2 dashboard.
Managing Infrastructure with Terraform
1. Update Resources
Modify the main.tf
file to reflect the desired changes. For example, change the instance type:
instance_type = "t3.micro"
Run the following commands:
terraform plan
terraform apply
2. Destroy Resources
To remove all resources defined in your configuration:
terraform destroy
This is useful for cleaning up resources after testing.
3. Use Variables
Variables allow you to parameterize configurations:
- Define variables in a
variables.tf
file:variable "region" { default = "us-east-1" }
- Reference variables in
main.tf
:provider "aws" { region = var.region }
- Pass variables at runtime:
terraform apply -var "region=us-west-2"
4. Manage State Files
Terraform maintains a state file (terraform.tfstate
) to track infrastructure changes. Store the state file securely to avoid inconsistencies.
To store the state file remotely, use a backend like S3:
terraform {
backend "s3" {
bucket = "my-terraform-state"
key = "state/terraform.tfstate"
region = "us-east-1"
}
}
Run terraform init
after configuring a backend.
Best Practices
- Use Version Control: Store your configuration files in a Git repository.
- Separate Environments: Create separate directories for staging, production, etc., with their own configurations.
- Lock Provider Versions: Specify exact provider versions in your configuration to ensure consistency:
provider "aws" { version = "~> 3.0" region = "us-east-1" }
- Validate Configurations: Use
terraform validate
to check for syntax errors. - Use Workspaces: Separate environments logically using Terraform workspaces:
terraform workspace new staging terraform workspace select staging
Conclusion
Terraform simplifies infrastructure management by treating it as code. By installing Terraform, writing declarative configurations, and adhering to best practices, you can automate and streamline resource provisioning across diverse platforms. Whether you’re managing a small cloud setup or a complex multi-cloud architecture, Terraform provides the tools needed for efficient and consistent infrastructure management.