How to Use bc for Basic Calculations in Shell
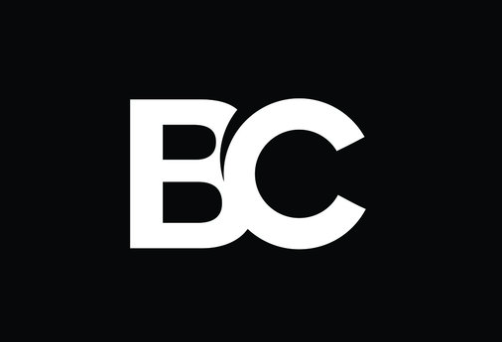
How to Use bc for Basic Calculations in Shell
When working in a Linux shell, performing calculations directly can be a daunting task, especially if you’re used to graphical interfaces. However, the command-line tool bc (short for “basic calculator”) simplifies this process. This article will guide you through the functionalities of bc, showcasing how to utilize it for basic calculations, making it a valuable addition to your command-line toolkit.
What is bc?
bc is an arbitrary precision calculator language that allows you to perform mathematical operations directly from the command line. It supports both basic arithmetic and advanced mathematical functions, making it a versatile tool for developers, system administrators, and anyone who frequently works in a terminal environment.
Installing bc
Most Linux distributions come with bc pre-installed. To check if it’s available on your system, simply open your terminal and type:
bc –version
If you don’t have it installed, you can easily install it using your package manager. For instance:
On Ubuntu/Debian:
sudo apt-get install bc
On CentOS/Fedora:
sudo dnf install bc
Once installed, you’re ready to start performing calculations.
Starting with bc
To use bc, simply type bc in your terminal and press Enter. You will see a prompt indicating that you’re in bc mode, ready to perform calculations.
$ bc
You can exit bc by typing quit or pressing Ctrl + D.
Basic Arithmetic Operations
Addition, Subtraction, Multiplication, and Division
The syntax for basic arithmetic operations in bc is straightforward. Here’s how to perform addition, subtraction, multiplication, and division:
- Addition: 2 + 3 evaluates to 5.
- Subtraction: 10 – 4 evaluates to 6.
- Multiplication: 7 * 8 evaluates to 56.
- Division: 20 / 4 evaluates to 5.
You can enter these operations directly into the bc prompt:
$ bc
2 + 3
5
10 – 4
6
7 * 8
56
20 / 4
5
Handling Division Precision
By default, bc performs integer division, meaning it truncates the decimal. If you want to perform floating-point division, you need to set the scale (number of digits after the decimal point). For example, to get two decimal places:
$ bc
scale=2
5 / 3
1.66
Parentheses for Order of Operations
Just like in standard arithmetic, you can use parentheses to dictate the order of operations. For example:
$ bc
(1 + 2) * (3 + 4)
21
Advanced Operations
Mathematical Functions
bc also supports a variety of built-in mathematical functions. Here are a few examples:
Square Root: Use the sqrt function to find the square root.
$ bc
sqrt(16)
4
Exponential and Logarithm: You can calculate exponential values using e (Euler’s number) and logarithms.
$ bc
e(1) # Euler’s number to the power of 1
2.718281828459045
l(10) # Natural logarithm of 10
2.302585092994046
Variables and Assignments
You can store values in variables for more complex calculations. For instance:
$ bc
x = 10
y = 20
x + y
30
This feature allows you to build more complex equations and perform repeated calculations without re-entering values.
Using bc in Command Line
You can also use bc directly from the command line without entering its interactive mode. For example, if you want to calculate 15 + 25, you can use:
echo “15 + 25” | bc
This command will output 40.
Redirecting Input from a File
If you have a file with multiple calculations, you can redirect input from the file into bc:
bc < calculations.txt
This command will execute all the calculations written in calculations.txt.
Scripting with bc
bc can be incorporated into shell scripts, making it a powerful tool for automating calculations in various scripts. Here’s a simple example of a script that calculates the area of a circle:
#!/bin/bash
read -p “Enter the radius: ” radius
area=$(echo “scale=2; 3.14 * $radius^2” | bc)
echo “The area of the circle is: $area”
This script prompts the user for a radius and calculates the area, displaying the result.