How to Set Up a Redis Cache for Your Web Application
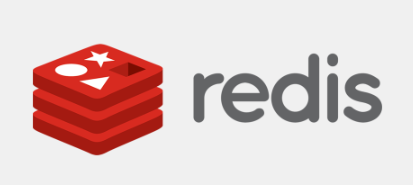
How to Set Up a Redis Cache for Your Web Application
In today’s fast-paced digital environment, web applications must be fast, responsive, and capable of handling a large number of requests efficiently. Caching plays a vital role in improving the performance of web applications, and one of the most popular caching solutions available is Redis. Redis, an in-memory data structure store, can be used as a cache, database, and message broker. This article will guide you through the steps to set up Redis as a cache for your web application.
Understanding Redis
Before we dive into the setup process, it’s important to understand what Redis is and how it works. Redis is an open-source, in-memory key-value store known for its high performance and flexibility. It supports various data structures, such as strings, hashes, lists, sets, and sorted sets, making it an ideal choice for caching dynamic web applications.
By caching frequently accessed data, Redis reduces the number of calls to your primary database, improving response times and reducing server load. This is particularly beneficial for read-heavy applications, where the same data is often retrieved multiple times.
Prerequisites
Before setting up Redis, ensure that you have the following:
- A web application: This can be built using any framework (e.g., Node.js, Django, Ruby on Rails).
- Redis installed on your server: You can install Redis on your local machine, a virtual private server (VPS), or any cloud service provider like AWS, Google Cloud, or Azure.
- To install Redis, follow the official installation guide: Redis Installation Guide.
Step 1: Install the Redis Client
Once Redis is installed, you need a Redis client for your application. Most programming languages have libraries that can interact with Redis. Here are some popular options:
- Node.js: node-redis
- Python: redis-py
- Ruby: redis-rb
- Java: Jedis
Install the appropriate library for your programming language. For example, if you’re using Node.js, run the following command:
npm install redis
For Python, you can use pip:
pip install redis
Step 2: Connecting to Redis
After installing the Redis client, the next step is to connect your web application to the Redis server. Here’s how to do this for different programming languages:
- Node.js Example
const redis = require(‘redis’);
const client = redis.createClient({
host: ‘localhost’, // Redis server hostname
port: 6379, // Redis server port
});client.on(‘connect’, () => {
console.log(‘Connected to Redis’);
});
- Python Example
import redis
client = redis.Redis(host=’localhost’, port=6379, db=0)
try:
client.ping()
print(“Connected to Redis”)
except redis.ConnectionError:
print(“Could not connect to Redis”)
- Ruby Example
require ‘redis’
redis = Redis.new(host: ‘localhost’, port: 6379)
begin
redis.ping
puts “Connected to Redis”
rescue Redis::CannotConnectError
puts “Could not connect to Redis”
end
Step 3: Caching Data
Now that you have established a connection to Redis, you can start caching data. Here are some common caching patterns:
Caching Database Queries
When your web application performs a database query, check if the result is already cached in Redis. If it is, return the cached result; otherwise, execute the query and store the result in Redis.
Here’s an example in Node.js:
const getData = async (key) => {
// Check cache
const cachedData = await client.get(key);
if (cachedData) {
return JSON.parse(cachedData); // Return cached data
}// If not in cache, perform the database query
const data = await databaseQuery(key); // Replace with your DB query
// Cache the result
client.setex(key, 3600, JSON.stringify(data)); // Cache for 1 hour
return data;
};
Caching API Responses
For API calls, you can cache the response in Redis similarly. This is particularly useful for third-party APIs with rate limits. Here’s an example in Python:
def get_api_data(api_url):
cache_key = f”api_cache:{api_url}”
cached_data = client.get(cache_key)
if cached_data:
return json.loads(cached_data)
response = requests.get(api_url)
data = response.json()
client.setex(cache_key, 3600, json.dumps(data)) # Cache for 1 hour
return data
Step 4: Expiration and Eviction Policies
One of the key features of Redis is the ability to set expiration times for cached data. This ensures that stale data does not persist indefinitely. You can use the EXPIRE command to set a time-to-live (TTL) for cached items. Additionally, Redis has various eviction policies to manage memory effectively when the cache fills up.
For example, you can set a key to expire in 60 seconds like this:
client.setex(‘key’, 60, ‘value’);
You can also configure eviction policies in your Redis configuration file (redis.conf). The most common policies include:
- volatile-lru: Evicts keys with an expiration set, using the least recently used (LRU) algorithm.
- allkeys-lru: Evicts any key using the LRU algorithm.
- volatile-random: Randomly evicts keys with an expiration set.
Thank you for visiting our article. Feel free to check out the links below to explore more Linux-related content and read our article on Wayland.
How to Install and Use Wayland on Ubuntu
To safely test the packages mentioned in our articles, visit our site through the link below, where you can rent servers tailored to your needs and create a reliable testing environment. Happy testing! 🙂